Deploying Llama 3.1 on AWS Cloud using AWS Bedrock: A Comprehensive Guide
Introduction
AWS Bedrock is a fully managed service that provides easy access to high-performing foundation models (FMs) from leading AI companies. This guide will walk you through the process of deploying Llama 3.1, a state-of-the-art large language model, on AWS Cloud using Bedrock.
Prerequisites
An AWS account with appropriate permissions
Basic familiarity with AWS services
AWS CLI installed and configured on your local machine
Python 3.7 or later installed
Step 1: Set Up AWS Bedrock
Log in to your AWS Management Console.
Navigate to the AWS Bedrock service.
If it's your first time using Bedrock, you may need to request access. Follow the prompts to do so.
Step 2: Choose Llama 3.1 Model
In the Bedrock console, go to the "Model playground" section.
Find and select "Llama 3.1" from the list of available models.
Step 3: Configure the Model
In the model configuration page, you can adjust various parameters such as:
Maximum token length
Temperature
Top-p value
Frequency penalty
Presence penalty
Customize these settings based on your specific use case.
Step 4: Create an API Key
In the Bedrock console, navigate to the "API keys" section.
Click "Create API key" and follow the prompts.
Save the API key securely; you'll need it to authenticate your requests.
Step 5: Set Up Your Python Environment
Create a new Python virtual environment
python -m venv llama3_env source llama3_env/bin/activate # On Windows, use `llama3_env\Scripts\activate`
Install the required libraries:
pip install boto3 requests
Step 6: Write Python Code to Interact with Llama 3.1
Create a new Python file (e.g., llama3_bedrock.py) and add the following code:
import boto3 import json bedrock = boto3.client( service_name='bedrock-runtime', region_name='your-region' # e.g., 'us-west-2' ) def generate_text(prompt, max_tokens=100): body = json.dumps({ "prompt": prompt, "max_tokens": max_tokens, "temperature": 0.7, "top_p": 0.95, }) response = bedrock.invoke_model( body=body, modelId='anthropic.llama-3-1' # Adjust if the actual model ID is different ) response_body = json.loads(response['body'].read()) return response_body['completion'] # Example usage prompt = "Explain the concept of quantum computing in simple terms." result = generate_text(prompt) print(result)
Step 7: Run Your Code
Execute your Python script
python llama3_bedrock.py
This will send a request to the Llama 3.1 model through AWS Bedrock and print the generated response.
Step 8: Monitor and Optimize
Use AWS CloudWatch to monitor your Bedrock usage, including:
API calls
Latency
Error rates
Analyze these metrics to optimize your implementation and manage costs.
Step 9: Implement Error Handling and Retries
Enhance your code to handle potential errors and implement retries
<pre>
import boto3 import json from botocore.exceptions import ClientError from time import sleep def generate_text_with_retry(prompt, max_tokens=100, max_retries=3): for attempt in range(max_retries): try: return generate_text(prompt, max_tokens) except ClientError as e: if e.response['Error']['Code'] == 'ThrottlingException': if attempt < max_retries - 1: sleep(2 ** attempt) # Exponential backoff continue raise raise Exception("Max retries reached")
</pre>
Step 10: Implement Caching (Optional)
To reduce API calls and improve response times, consider implementing a caching mechanism:
import hashlib cache = {} def generate_text_cached(prompt, max_tokens=100): cache_key = hashlib.md5(f"{prompt}:{max_tokens}".encode()).hexdigest() if cache_key in cache: return cache[cache_key] result = generate_text(prompt, max_tokens) cache[cache_key] = result return result
Conclusion
You've now successfully set up and deployed Llama 3.1 on AWS Cloud using Bedrock. This powerful combination allows you to leverage state-of-the-art language models with the scalability and reliability of AWS infrastructure.
Remember to keep your API keys secure, monitor your usage, and optimize your implementation as needed.
As you continue to work with Llama 3.1 and AWS Bedrock, explore advanced features such as fine-tuning the model for specific tasks, implementing streaming responses for real-time applications, and integrating the model with other AWS services for more complex AI-powered solutions.
Services
Professional consulting services company providing AI/ML services for industry.
About SOHAMLABS
Resources
Careers
© 2024. All rights reserved.

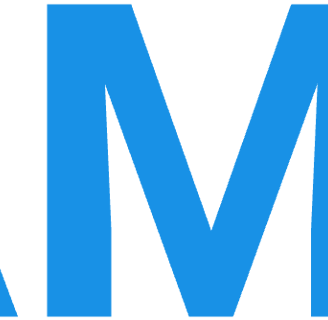
Blogs
Customers
Services
Solutions